So, people sometimes ask how I got decent at handling web cookies, enough that a buddy jokingly called me the “cookie king” once. It wasn’t exactly planned, more like trial by fire, really.
It all started when I was trying to automate some stuff online. Just simple things, grabbing data from a few sites, maybe clicking a button here and there. Nothing fancy. But man, the login sessions were a nightmare. I’d get my script working, leave it running, and boom – logged out. Back to square one. Every single time.
The First Messy Attempts
Naturally, I did what anyone would do. I tried saving the cookies after logging in with one script, then loading them in another. Sometimes it worked, sometimes it didn’t. It felt completely random.
Here’s what I tried initially:
- Using built-in browser tools to export cookies. Super manual, useless for automation.
- Basic libraries in Python, like Requests’ session object. Better, but still flaky across different sites or if I stopped and started the script.
- Trying to manually copy-paste cookie values from browser developer tools into my scripts. Yeah, that lasted about five minutes.
It was just a big tangled mess. Each website seemed to have its own quirks. Some cookies expired quickly, others needed specific headers sent along, and keeping track of it all, especially when running multiple scripts, was just painful. I spent more time debugging login issues than actually getting the work done.
Figuring Things Out
The turning point came when I stopped treating cookies like these magic tokens and started thinking about them simply as the website’s way of remembering my browser. If I wanted my scripts to be remembered, they needed to present the exact same memory the website expected.
So, I decided I needed one central place to keep all the cookies, like a shared cookie jar. I started experimenting:
- First, I tried using a simple text file. Log in with one script, dump all the cookies into the file. Another script reads the file before making requests.
- This was better, more reliable. I made sure to handle file locking, so scripts wouldn’t mess up the file reading or writing it at the same time.
- Then I refined it. Instead of just dumping everything, I made sure to update cookies correctly. If the site sent back a `Set-Cookie` header, my script would update the jar file.
It wasn’t rocket science, just persistence. I wrote some simple helper functions to load cookies from my jar before making a request and save them back after. I made sure user-agent strings and other headers matched what the browser sent during the initial login.
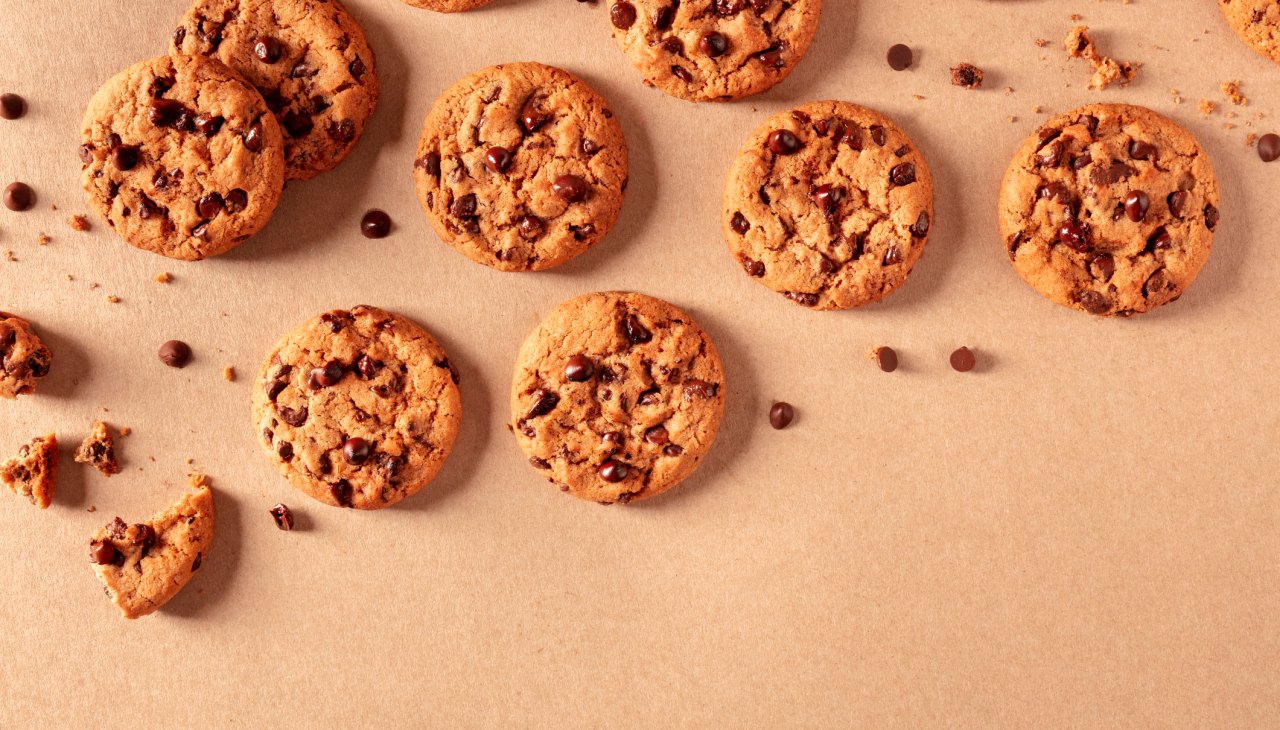
Becoming the So-Called “King”
Suddenly, things started working. My scripts stayed logged in for days, sometimes weeks. I could run different scripts targeting the same site, and they all shared the same login session seamlessly because they were all using the same cookie jar. It felt like I’d finally cracked it.
The key wasn’t some complex algorithm; it was just being meticulous:
- Centralize cookie storage (a file worked fine for my scale).
- Ensure cookies are loaded before requests.
- Ensure cookies are saved after responses (if the site sends new ones).
- Keep other request details (like User-Agent) consistent.
That’s pretty much it. No magic library, no secret sauce. Just understanding the basic flow and sticking to it rigorously. It made my automation tasks way less frustrating. So yeah, “cookie king” is a stretch, but I definitely tamed those pesky browser biscuits for my own needs. It was born out of pure necessity and a lot of annoying failures.